Model Context Protocol (MCP) Explained
Model Context Protocol (MCP): Connecting AI Models to Real-World Data
Connecting powerful language models to real-world data and the systems they require has consistently been a challenge. Even the most advanced AI models often operate in isolation, cut off from the vital context needed to perform effectively. This disconnect has resulted in fragmented integrations, data silos, and complex workflows, hindering the application of AI in real-world scenarios.
In November 2024, Anthropic introduced an open standard called the Model Context Protocol (MCP). This protocol is designed to change how AI models interact with external tools and data sources, aiming to resolve the complex problem of connecting multiple AI models with numerous data sources and tools—a longstanding issue for enterprises and developers.
This guide explains what MCP is and how it works, details its architecture, explains how you can get started with MCP, and discusses benefits and challenges to consider.
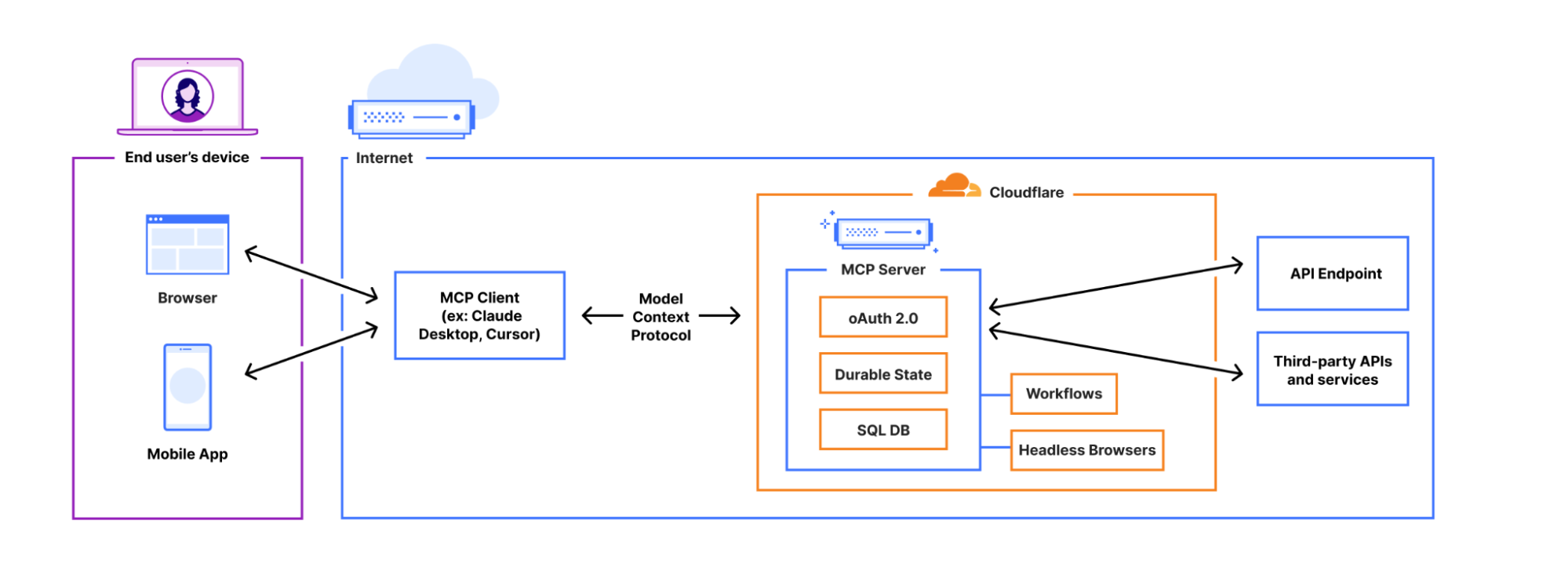
What is the Model Context Protocol?
The Model Context Protocol (MCP) is an open standard developed by Anthropic that offers a universal method for connecting AI assistants to external data sources and systems. It addresses the challenge of integrating AI models with diverse tools, databases, and content repositories in a standardized manner.
MCP serves as a universal interface that enables AI models to interact with external systems, ranging from cloud platforms like GitHub and Slack to enterprise databases and local files, without requiring custom integrations for each new data source or tool.
At its core, MCP addresses what’s known as the “M×N integration problem,” which refers to the exponential complexity of connecting M AI models to N tools or data sources. Instead of building one-off connectors for every combination, MCP provides a standardized protocol that allows any AI application (like Claude Desktop or an IDE) to communicate with any compliant data source or service.
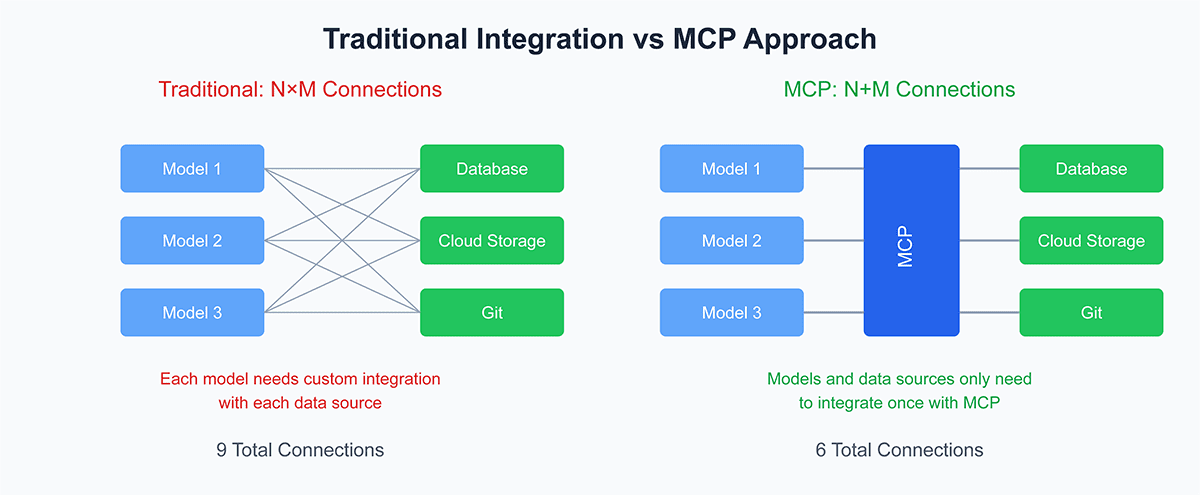
Core Principles of MCP
Universal Connectivity
MCP acts as an “AI USB port”, allowing for seamless integration between language models and external systems. Whether accessing Google Drive files, querying Postgres databases, or automating GitHub workflows, MCP provides a consistent interface for AI to retrieve data and perform actions.
Structured Context Management
Unlike traditional methods like RAG or monolithic prompts, MCP organizes interactions into three standardized primitives:
- Tools: Executable functions (e.g., API calls, database queries)
- Resources: Structured data streams (e.g., files, logs, API responses)
- Prompts: Reusable instruction templates for common workflows
Local-First Security
MCP prioritizes privacy by default. This means it requires explicit user approval for every tool or resource access. Servers run locally unless explicitly permitted for remote use, so sensitive data won’t leave controlled environments without consent.
How Does the MCP Work?
- Connection Establishment: An MCP host (explanation below) initiates a connection to one or more MCP servers.
- Capability Discovery: The MCP client queries the server to discover available tools, resources, and prompts.
- Context Augmentation: When a user interacts with the AI model, the host enriches the model's context with relevant information from connected MCP servers.
- Tool Selection and Execution: Based on the user's query and available tools, the AI model decides which MCP tools to use. The MCP client then executes these tools via the appropriate server.
- Response Generation: The AI model incorporates the results from MCP servers into its response, providing more accurate and contextually relevant answers.
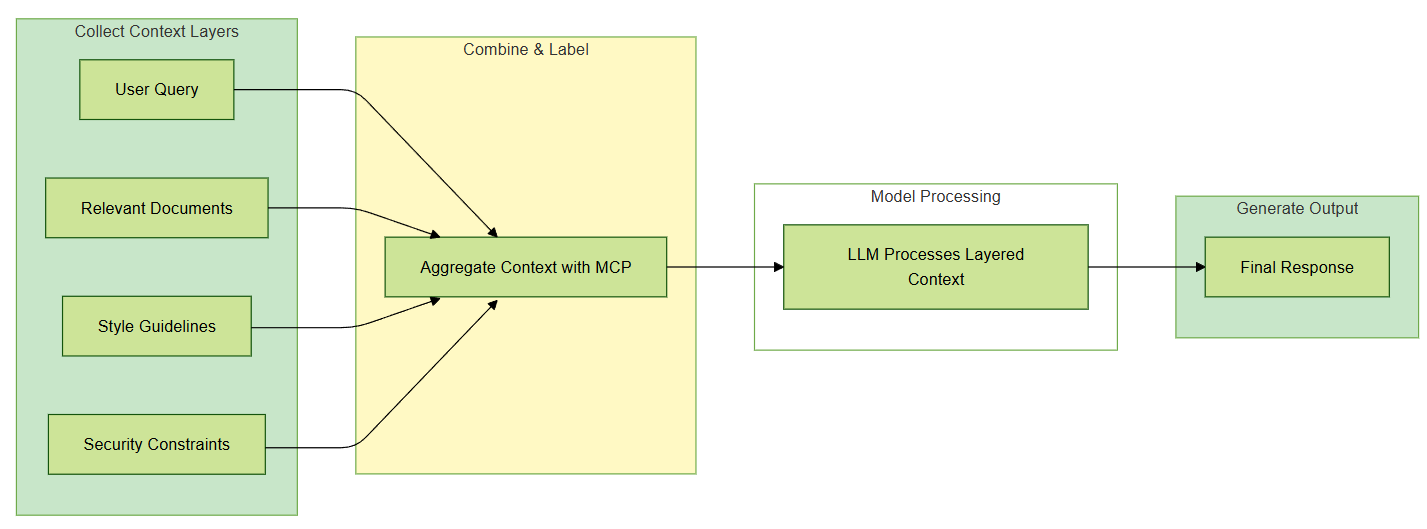
MCP Architecture
MCP is made up of three core building blocks:
- Host: Hosts are the central AI-powered applications (like Claude Desktop or Cursor) that users interact with directly. They serve as the primary interface for AI functionality and manage the overall system.
- Clients: Clients act as intermediaries, maintaining individual connections between the host application and MCP servers. They handle the communication protocol and manage the flow of data and commands.
- Servers: Servers are specialized components that expose specific functionalities, data sources, or tools to AI models through a standardized interface.
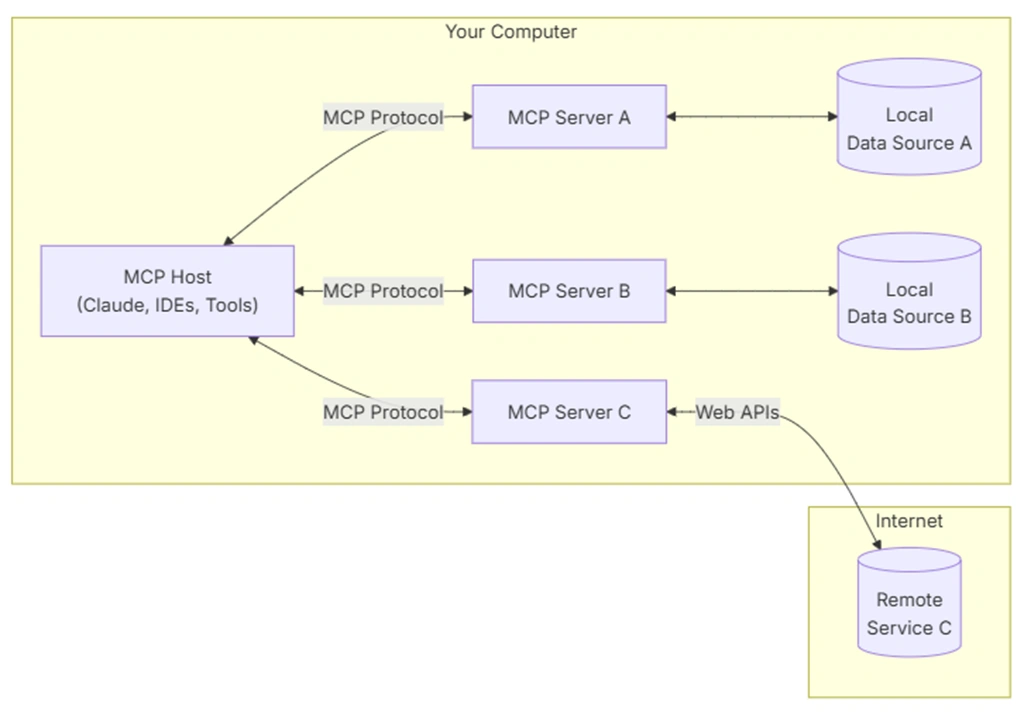
Getting Started with MCP
The MCP provides a standardized way for AI assistants to connect with external data sources and tools. Let's look at the process of building an MCP server, from initial setup to connecting it with Claude.
Introduction for Server Developers
Building an MCP server lets your enterprise extend your AI assistants' capabilities by providing them access to external data sources and tools through a standardized interface. Let’s look at creating a simple weather server that can be queried by Claude or other MCP-compatible clients.
1. Set Up the Environment
Install necessary tools and create a new Python project with required dependencies.
curl \-LsSf https://astral.sh/uv/install.sh | sh
uv init weather
cd weather
uv venv
source .venv/bin/activate
uv add "mcp\[cli\]" httpx
touch weather.py
2. Define the Server
Use the FastMCP
class to initialize an MCP server.
from mcp.server.fastmcp import FastMCP
# Initialize FastMCP server
mcp = FastMCP("weather")
3. Implement Tool Logic
Create functions that perform the desired tasks, such as fetching weather data from an API.
@mcp.tool()
async def get_forecast(latitude: float, longitude: float) -> str:
"""Get weather forecast for a location."""
# Implementation details...
4. Run the Server
Use the mcp.run() method to start the server with the appropriate transport.
if __name__ == "__main__":
# Initialize and run the server
mcp.run(transport='stdio')
5. Connect to a Client
Configure an MCP client (like Claude for Desktop) to use the server.
{
"mcpServers": {
"weather": {
"command": "uv",
"args": [
"--directory",
"/ABSOLUTE/PATH/TO/PARENT/FOLDER/weather",
"run",
"weather.py"
]
}
}
}
How to Build MCPs with LLMs
Building MCP servers can be significantly accelerated by leveraging LLMs like Claude to generate code and solve implementation challenges. This lets developers focus on defining requirements instead of wrestling with complex protocol details and boilerplate code.
1. Gather Documentation
- Gather comprehensive MCP documentation from modelcontextprotocol.io/llms-full.txt
- Collect SDK documentation from the Python or TypeScript repositories
- Provide these materials to Claude or another frontier LLM to establish context
2. Define Server Requirements
- Clearly describe the server's purpose and functionality
- Specify which resources, tools, and prompts your server will provide
- Identify external systems it needs to interact with (databases, APIs, etc.)
- Example:
Build an MCP server that connects to PostgreSQL, exposes schemas as resources, and provides SQL query tools
3. Iterative Development with LLM
- Start with core functionality, then expand
- Ask the LLM to explain unclear code sections
- Request modifications and improvements as needed
- Use the LLM for testing and edge case handling
4. Implement Key MCP Features
- Resource management and exposure
- Tool definitions and implementations
- Prompt templates and handlers
- Error handling and logging
- Connection and transport setup
5. Test and Refine
- Have the LLM help write test cases for your server
- Request improvements based on test results
- Iterate on specific features until they work as expected
6. Finalize and Deploy
- Review the generated code thoroughly
- Use the MCP Inspector tool to test your server
It’s also worth noting that using LLMs as a judge can provide a cost-effective way to evaluate MCP server outputs at scale. Note that you’ll need to take care to validate the evaluator's performance.
How to Debug Integrations With MCP
When it comes to troubleshooting MCP integrations, you’ll need a systematic approach to identify and resolve issues that may arise when connecting AI models with external data sources. Effective LLM monitoring practices are critical for identifying issues early and maintaining high-quality AI performance when using MCP in production environments.
1. Check Server Status in Claude Desktop
First, verify your MCP servers are properly connected:
- Click the 🔨 icon to view available tools
- Check that your tools appear in the list (like get-forecast and get-alerts for a weather server)
- If tools aren't showing up, check your configuration file:
2. View MCP Logs
Access detailed MCP logs to identify issues:
# Follow logs in real-time
tail -n 20 -F ~/Library/Logs/Claude/mcp*.log
These logs capture connections with servers, configuration issues, runtime errors, and message exchanges.
3. Enable Developer Tools
For deeper inspection, enable Chrome DevTools:
# Create developer settings file
echo '{"allowDevTools": true}' > ~/Library/Application Support/Claude/developer_settings.json
Then open DevTools using Command-Option-Shift-i and use:
- Console panel to check for JavaScript errors
- Network panel to inspect message payloads
4. Fix Common Issues
For working directory problems, you should always use absolute paths in your configuration:
{
"command": "npx",
"args": [
"-y",
"@modelcontextprotocol/server-filesystem",
"/Users/username/data"
]
}
You'll also want to provide necessary environment variables in your configuration:
{
"myserver": {
"command": "mcp-server-myapp",
"env": {
"MYAPP_API_KEY": "some_key"
}
}
}
5. Add Logging to Your Server
Implement proper logging in your server code:
server.request_context.session.send_log_message(
level="info",
data="Server started successfully",
)
Troubleshooting MCP integrations requires a systematic approach to identify and resolve issues that may arise when connecting AI models with external data sources. To fully leverage the capabilities of MCP, it's crucial to understand techniques for maximizing LLMs through effective integration and context management.
Benefits of MCP
1. Standardized Integration
MCP provides a universal method for connecting AI models to various data sources and tools. This way, you won’t need custom integrations for each new data source or tool, significantly reducing development time and complexity.
For example, instead of writing separate connectors for GitHub, Slack, and Google Drive, you can use a single MCP-compliant interface to access all these services.
2. Enhanced Context Awareness
By letting AI models access real-time data from external sources, MCP dramatically improves their ability to provide relevant and up-to-date responses. This context awareness lets AI assistants draw on the most current information available instead of only relying on their training data.
For example, a customer support chatbot using MCP could access the latest product information, user account details, and company policies in real-time. That way, its responses are highly likely to be accurate and contextually appropriate.
3. Dynamic Tool Discovery and Execution
MCP introduces a powerful capability for AI models to dynamically discover and utilize available tools. Instead of being limited to a predefined set of functions, an AI using MCP can query for available tools at runtime and decide how to use them based on the current context. This flexibility allows for more adaptive and capable AI systems that can handle a wider range of tasks - all without requiring constant updates to their core functionality.
4. Improved Security and Access Control
Security is a core consideration in MCP's design. The protocol incorporates built-in authentication and access control mechanisms, so your AI models only access data and tools that they are explicitly authorized to use.
This local-first, permission-based approach allows organizations to maintain tight control over their data while still enabling powerful AI integrations. For example, an enterprise could use MCP to give an AI assistant access to specific internal databases without exposing sensitive information or granting unnecessary privileges.
5. Ecosystem Growth and Interoperability
As an open standard, MCP helps with the development of a rich ecosystem of compatible tools and services.
This interoperability means that as new MCP-compliant tools are developed, they can be immediately utilized by any AI system that supports the standard protocol. This ecosystem growth benefits both developers and end-users by continually expanding the capabilities of AI applications without requiring significant rework.
For instance, a coding assistant using MCP could easily incorporate new code analysis tools or version control systems as they become available, improving its functionality over time.
Challenges of MCP
While MCP offers tremendous potential for connecting AI models with external data sources, there are implementation challenges that should be considered.
1. Engineering Complexity and System Overhead
Introducing MCP means adding extra components to your enterprise architecture - an MCP server layer and client adapters across various systems. This additional complexity can introduce performance overhead since each tool call becomes an out-of-process remote procedure call (RPC) rather than a simple in-process function call.
In high-throughput enterprise environments, the constant serialization/deserialization and context-switching between systems could become a bottleneck if not properly optimized.
2. Scalability and Performance
As MCP adoption grows, ensuring consistent performance under heavy loads becomes crucial. Handling numerous simultaneous connections between AI models and data sources can strain system resources, potentially impacting overall performance. It makes it more difficult to ensure low-latency responses as the number of integrated systems increases, especially for real-time applications.
3. Standardization and Fragmentation
The emerging nature of MCP technology introduces risks of fragmentation if multiple competing standards develop. Different organizations may create incompatible versions of the protocol, leading to interoperability issues.
Proprietary extensions to MCP could result in vendor lock-in, limiting the flexibility of implementations. In addition, ensuring backward compatibility as the protocol evolves might become challenging, so there could potentially be barriers to upgrades.
4. Integration Complexity
While MCP aims to simplify integrations, it introduces its own learning curve and integration complexities. Enterprises need to familiarize themselves with MCP-specific concepts like prompts, resources, and tools. These may require additional training and expertise.
Moreover, implementing a comprehensive system for evaluating LLMs is essential when integrating AI models with external data sources via MCP to ensure consistent performance and reliability.
Validating MCP integrations across multiple systems demands sophisticated testing environments and methodologies. As a new technology, comprehensive guides and best practices are still evolving, so it’s quite possible that there are still some knowledge gaps.
5. Identity Management and Authentication
Identity management and authentication across different systems connected via MCP present another set of challenges. Additionally, different integrated systems may use varying authentication methods, so it complicates user management and access control.
Translating user permissions across multiple systems connected via MCP can be complex, requiring careful mapping and synchronization. Moreover, managing identities across organizational boundaries becomes more intricate in MCP scenarios. This is especially the case in multi-tenant or federated environments.
Learn More About MCP
The MCP offers a powerful solution for seamlessly connecting AI models to external data sources and tools, allowing for more context-aware and capable AI applications. Whether you're working with Claude, GPT, or other LLMs, MCP helps standardize integrations, reduce development time, and unlock new possibilities for AI-powered systems.
Humanloop is the LLM evals platform for enterprises. We enable product and engineering teams to adopt best practices for developing, deploying, and continuously improving AI applications and agents.
To explore how Humanloop can support your team in creating, managing, and refining AI integrations using MCP, book a demo today.
About the author
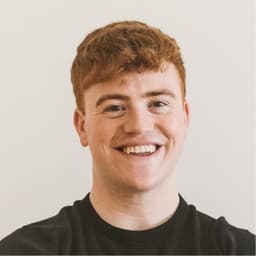
- 𝕏@conorkellyai
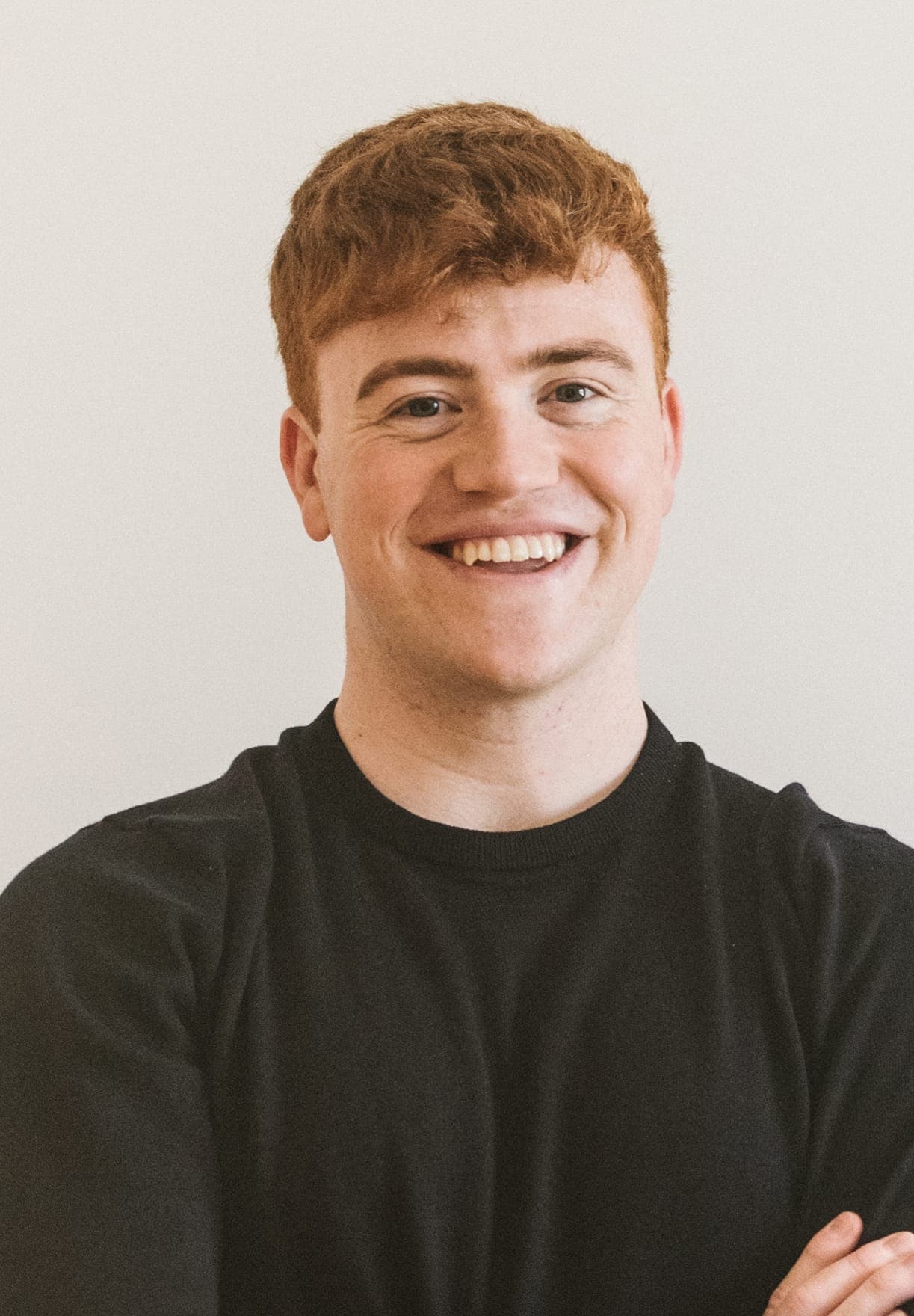
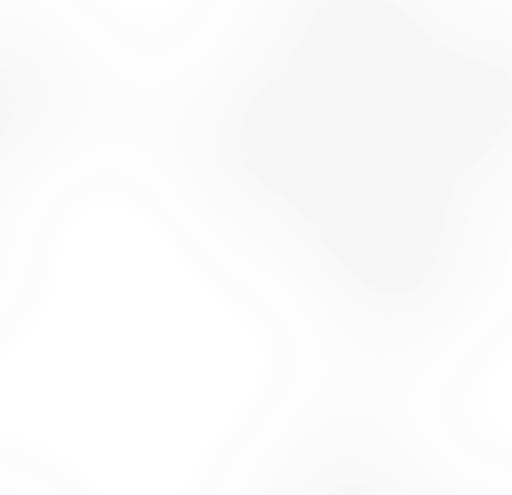
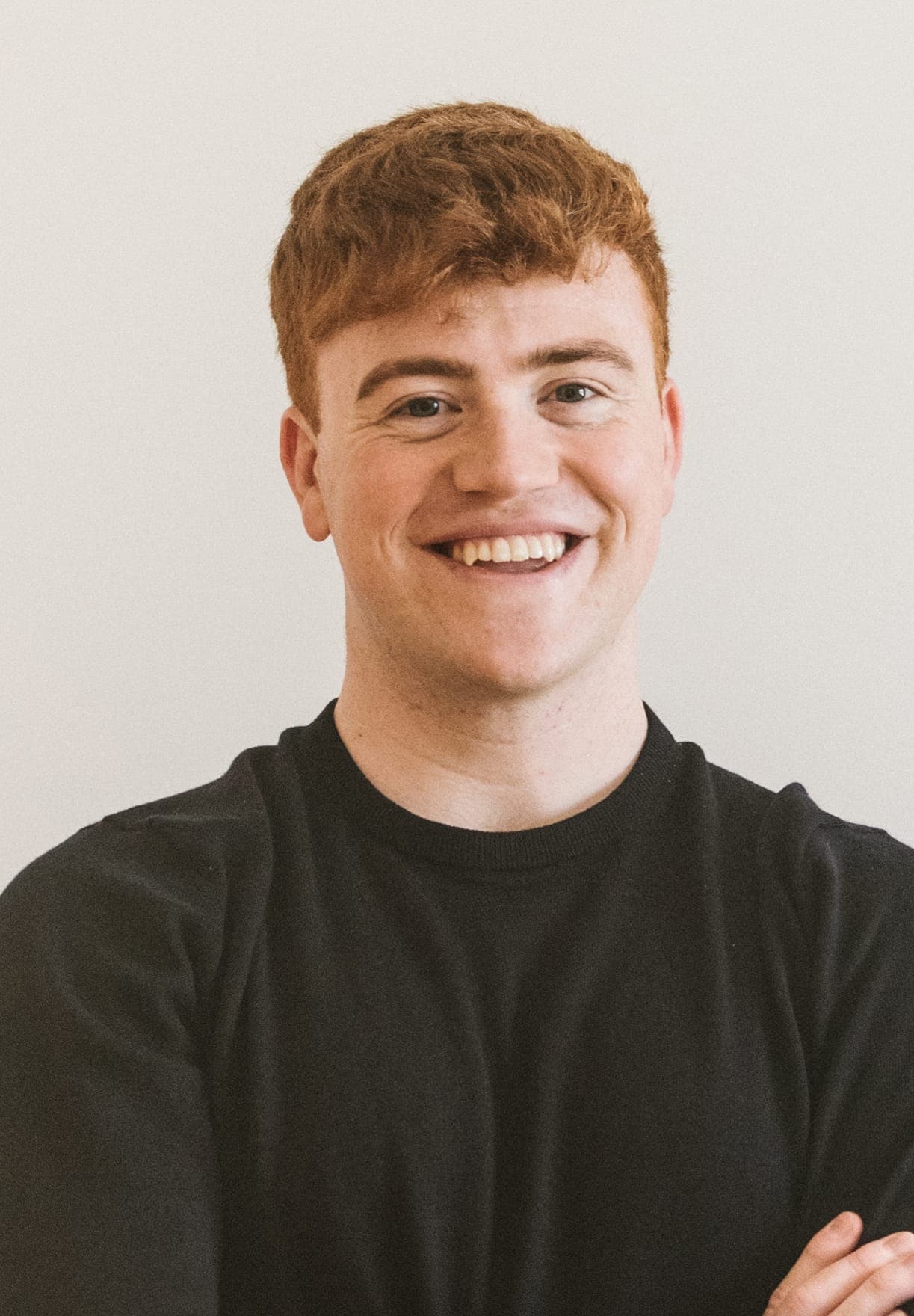