OpenAI Agents SDK
OpenAI’s Agents SDK: Explained
The OpenAI Agent SDK is a Python-based framework designed to simplify the development of agentic AI applications. Building upon OpenAI’s experimental Swarm project, the SDK introduces production-ready primitives that make it easy to create sophisticated workflows without a steep learning curve.
In this guide, we’ll look at how it works, how you can get started with it, along with various benefits and challenges you should keep in mind.
What is the OpenAI Agents SDK?
The OpenAI Agents SDK provides developers with a lightweight, production-ready framework for creating intelligent agents - AI models equipped with the ability to follow instructions, use tools, and delegate tasks to other agents.
Building on OpenAI’s earlier experimental project, Swarm, the SDK introduces a refined set of primitives that make it easy to build sophisticated, real-world AI workflows without a steep learning curve.
The SDK is designed with simplicity and flexibility in mind. It integrates seamlessly with Python’s native features, allowing developers to orchestrate complex workflows without needing to learn new abstractions. Additionally, it includes built-in tracing tools for debugging, monitoring, and visualizing agent flows, making it easier to understand how agents interact and make decisions.

How does the Agents SDK work?
The OpenAI Agents SDK introduces a structured approach for building scalable and reliable AI applications by leveraging four key components:
- Agents
- Handoffs
- Guardrails
- Tracing
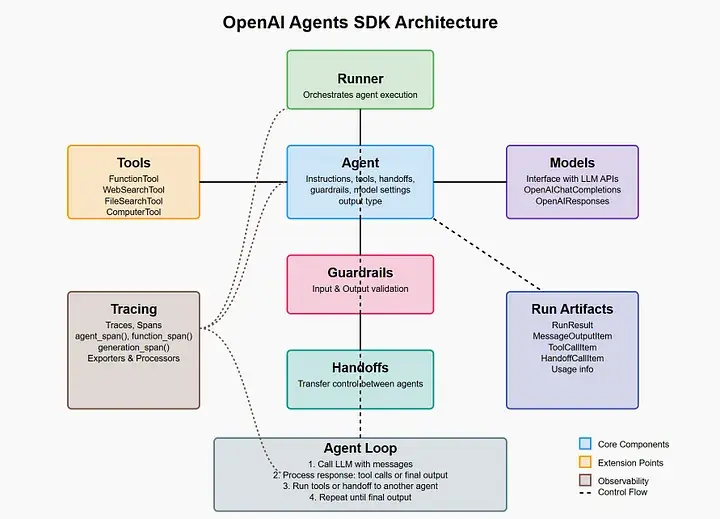
Agents
Agents are the core entities in the SDK, representing individual language models equipped with specific instructions and tools. Each agent is configured to perform a specialized role, such as answering questions, generating code, or retrieving information.
Agents work by combining predefined instructions with tool integrations that allow them to perform actions beyond text generation. For example, an agent can execute code, query databases, or search the web. This modular design provides flexibility, so enterprises create purpose-driven agents tailored to their application needs.
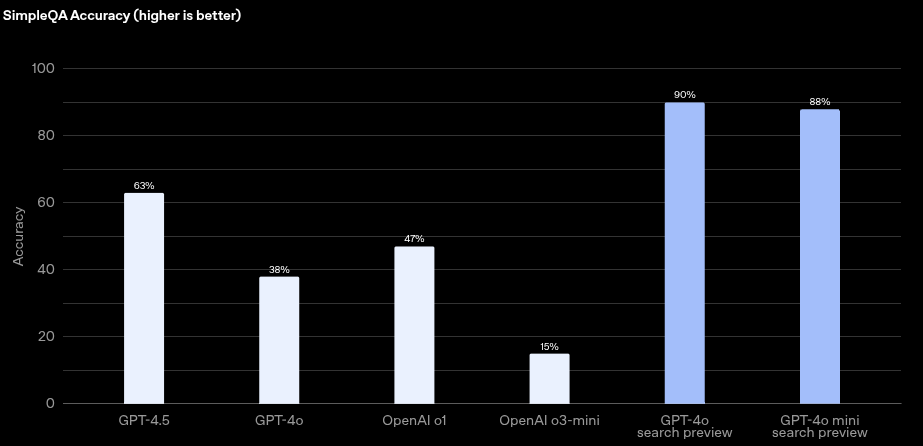
Handoffs
Handoffs help with seamless task delegation between agents based on their capabilities. When an agent encounters a query or task outside its scope, it can transfer the responsibility to another agent better suited for handling it.
This mechanism ensures efficient workflow orchestration by allowing multiple agents to collaborate dynamically. Handoffs are triggered based on predefined rules or contextual analysis performed by the SDK, ensuring that tasks are routed correctly without manual intervention.
Guardrails
Handoffs allow for seamless task delegation between agents based on their capabilities. When an agent encounters a query or task outside its scope, it can transfer the responsibility to another agent better suited for handling it.
This mechanism provides efficient workflow orchestration by allowing multiple agents to collaborate dynamically. Handoffs are triggered based on predefined rules or contextual analysis performed by the SDK, ensuring that tasks are routed correctly without manual intervention.
Tracing
Tracing offers built-in observability for debugging and monitoring agent workflows. It allows developers to visualize how agents interact with tools, handle queries, and make decisions during execution.
The tracing system provides detailed logs of tool usage, decision-making processes, and task delegation events. This transparency is invaluable for debugging complex workflows and ensuring compliance with organizational standards.
Getting Started with the Agents SDK
The OpenAI Agents SDK makes it easy to create intelligent, task-oriented AI agents that can interact with tools, delegate tasks, and validate inputs and outputs. This introductory guide walks you through setting up your environment, creating agents, defining workflows, and integrating guardrails.
1. Create a Project and Virtual Environment
Start by creating a project folder and setting up a virtual environment for your development.
mkdir my_project
cd my_project
python -m venv .venv
Activate the virtual environment every time you start a new terminal session:
source .venv/bin/activate
2. Install the Agents SDK
Install the OpenAI Agents SDK using pip:
pip install openai-agents
If you don’t have an OpenAI API key, follow OpenAI’s instructions to create one. Then set your API key:
export OPENAI_API_KEY=sk-...h
3. Create Your First Agent
Agents are defined with instructions, a name, and optional configurations like model settings.
Here's an example of creating a math tutor agent:
from agents import Agent
agent = Agent(
name="Math Tutor",
instructions="You provide help with math problems. Explain your reasoning at each step and include examples."
)
4. Add More Agents
You can define additional agents for specialized tasks, such as history tutoring or triage routing:
history_tutor_agent = Agent(
name="History Tutor",
handoff_description="Specialist agent for historical questions",
instructions="You provide assistance with historical queries. Explain important events and context clearly."
)
math_tutor_agent = Agent(
name="Math Tutor",
handoff_description="Specialist agent for math questions",
instructions="You provide help with math problems. Explain your reasoning at each step and include examples."
)
5. Define Handoffs
Handoffs allow one agent to delegate tasks to another based on predefined rules or contextual analysis. For example, a triage agent can route homework questions to the appropriate tutor:
triage_agent = Agent(
name="Triage Agent",
instructions="You determine which agent to use based on the user's homework question.",
handoffs=[history_tutor_agent, math_tutor_agent]
)
6. Run the Workflow
Use the Runner
class to execute workflows and verify that the triage agent correctly routes queries between specialist agents:
from agents import Runner
async def main():
result = await Runner.run(triage_agent, "What is the capital of France?")
print(result.final_output)
7. Add Guardrails
Guardrails ensure safe and validated operations by checking inputs or outputs against predefined rules. You can implement custom guardrails using Pydantic models:
from agents import GuardrailFunctionOutput, Agent, Runner
from pydantic import BaseModel
class HomeworkOutput(BaseModel):
is_homework: bool
reasoning: str
guardrail_agent = Agent(
name="Guardrail Check",
instructions="Check if the user is asking about homework.",
output_type=HomeworkOutput,
)
async def homework_guardrail(ctx, agent, input_data):
result = await Runner.run(guardrail_agent, input_data, context=ctx.context)
final_output = result.final_output_as(HomeworkOutput)
return GuardrailFunctionOutput(
output_info=final_output,
tripwire_triggered=not final_output.is_homework,
)
8. Combine Everything
Put all components together to create a workflow with handoffs and guardrails:
triage_agent = Agent(
name="Triage Agent",
instructions="You determine which agent to use based on the user's homework question.",
handoffs=[history_tutor_agent, math_tutor_agent],
input_guardrails=[
InputGuardrail(guardrail_function=homework_guardrail),
],
)
async def main():
result = await Runner.run(triage_agent, "Who was the first president of the United States?")
print(result.final_output)
if __name__ == "__main__":
asyncio.run(main())
9. View Your Traces
To debug workflows or review agent interactions, navigate to the Trace Viewer in the OpenAI Dashboard. This tool provides detailed logs of tool usage, decision-making processes, and task delegation events.
Benefits of Using the Agents SDK
1. Simplified Development Workflow
The OpenAI Agents SDK eliminates the complexity of building AI workflows by providing a Python-first design that integrates seamlessly into existing codebases. Enterprises can define agents with minimal boilerplate code, focusing on business logic rather than infrastructure.
It automates repetitive tasks such as tool execution and result processing, freeing developers from handling low-level orchestration. Moreover, the SDK provides intuitive primitives like agent, runner, and guardrails. Consequently, it makes it easy to build, test, and deploy workflows.
This simplicity is particularly valuable for teams transitioning from experimental AI models to production-grade systems, allowing for faster prototyping and deployment.
2. Multi-Agent Coordination
The SDK introduces dynamic handoffs, allowing multiple agents to collaborate seamlessly. Agents can delegate tasks based on predefined rules or contextual analysis, ensuring efficient workflow orchestration.
This means the SDK enables specialized agents to handle distinct tasks (e.g., customer support, technical debugging) while working together dynamically. It also prevents bottlenecks by routing tasks to the most capable agent automatically. In addition, it improves task resolution efficiency by leveraging the strengths of different agents.
3. Built-In Guardrails for Safety and Validation
The SDK includes robust guardrails that validate inputs and outputs in real time, ensuring safe and reliable operations. These safeguards are critical for enterprise applications where security and compliance are paramount.
This is seen by the fact that it prevents harmful or inappropriate outputs through moderation tools. Moreover, the SDK validates output formats using Pydantic-powered schema checks.
For instance, guardrails can filter user inputs for profanity or validate that responses conform to specific JSON schemas before they are returned to users. This ensures high-quality interactions while minimizing risks.
4. Scalability for Enterprise Applications
The SDK is engineered for high-throughput environments, making it suitable for large-scale deployments. It supports asynchronous execution and integration with external APIs and tools.
It also reduces operational costs by automating repetitive tasks like customer support or research assistance. Consequently, enterprises can ensure reliability in production environments through built-in load balancing mechanisms.
Challenges and Considerations
1. Limited Support for Non-OpenAI Models
While the SDK is optimized for OpenAI models, support for other LLM providers is limited. For instance, structured outputs like JSON schema validation may not be supported by alternative providers, leading to errors when attempting to use tools that rely on structured outputs.
Developers working with non-OpenAI models may face issues such as 404 errors or malformed JSON outputs due to missing support for structured outputs or tool integration. The SDK’s reliance on OpenAI-specific features like the responses API can restrict flexibility when integrating third-party LLMs.
2. Complexity in Multi-Agent Orchestration
While the SDK simplifies agent creation and handoffs, orchestrating workflows involving multiple agents can become complex as tasks scale. Managing inter-agent communication, task delegation logic, and context sharing requires careful design and debugging.
It’s challenging because multi-agent systems require precise handoff rules and context management to ensure seamless collaboration. Misconfigured handoffs can lead to agents failing to delegate tasks correctly or producing incomplete results.
Debugging workflows involving multiple agents can be time-consuming, especially when tracing errors across different handoffs or guardrails.
3. Tracing and Observability Limitations
The SDK provides built-in tracing capabilities to monitor workflows, but these features are heavily reliant on OpenAI’s infrastructure. Developers without an OpenAI API key may encounter issues uploading traces or accessing trace data.
Tracing errors (e.g., client error 401) occur if API keys aren’t properly configured for tracing uploads, forcing developers to disable tracing entirely or use alternative processors. In addition, limited support for non-OpenAI trace processors can hinder observability in multi-provider environments.
Without robust tracing, debugging workflows becomes more difficult, especially for enterprise applications requiring detailed audit trails. To address the limitations in tracing and observability within the OpenAI Agents SDK, particularly for developers seeking a model-agnostic alternative, consider integrating Humanloop into your workflow.
Humanloop offers a comprehensive platform for LLM observability, designed to be accessible to both technical and non-technical users. It provides comprehensive tools for monitoring model performance, user behavior, and system health in real time.
4. Collaboration Across Teams
The OpenAI Agents SDK’s technical design primarily targets engineers, creating barriers for non-technical stakeholders like product managers and domain experts who need to contribute to agent development.
Configuring agents requires Python expertise and familiarity with concepts like YAML headers, Pydantic schemas, and async workflows-skills often limited to engineering teams. It’s also worth noting that on-technical users struggle to review or contribute to agent definitions stored in code repositories, leading to misaligned expectations.
Product managers and domain experts cannot directly edit prompts, tools, or guardrails. As a result, it forces engineers to act as intermediaries and slows iteration cycles.
Learn More
The OpenAI Agents SDK empowers developers to create intelligent, scalable AI systems with ease. By providing a Python-first framework for building agentic workflows, the SDK simplifies development, enhances multi-agent coordination, and ensures safety through built-in guardrails. With powerful tracing tools and enterprise-grade scalability, it’s the ideal solution for teams looking to transition from prototypes to production-ready AI applications.
At Humanloop, we help enterprises implement cutting-edge AI solutions, including agent-based workflows powered by the OpenAI Agents SDK. Our platform provides the tools you need to streamline development, optimize performance, and ensure compliance across complex AI systems.
To learn more about how the OpenAI Agents SDK and Humanloop’s enterprise-grade AI development platform can accelerate your team’s workflow, book a demo today.
About the author
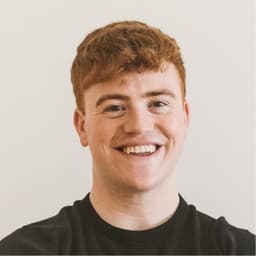
- 𝕏@conorkellyai
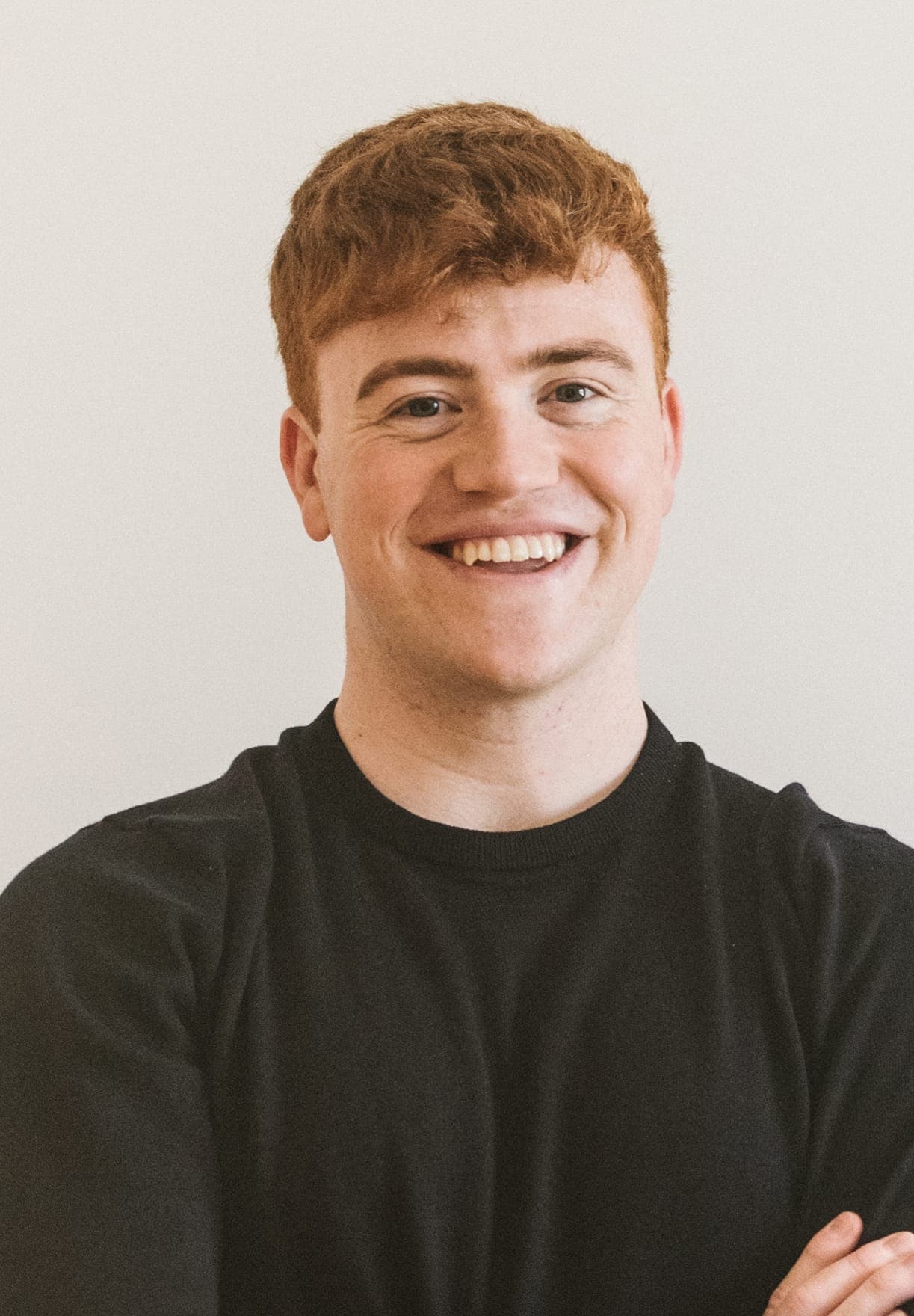
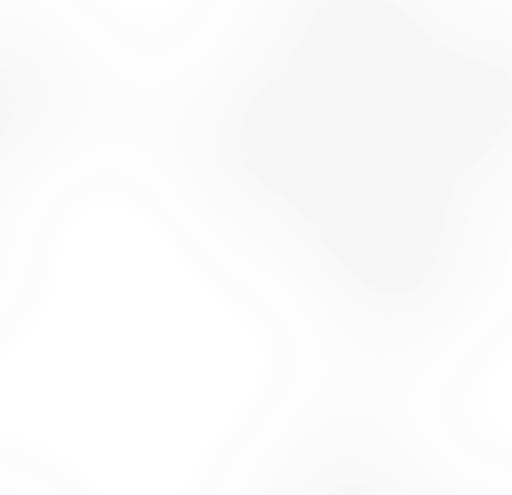
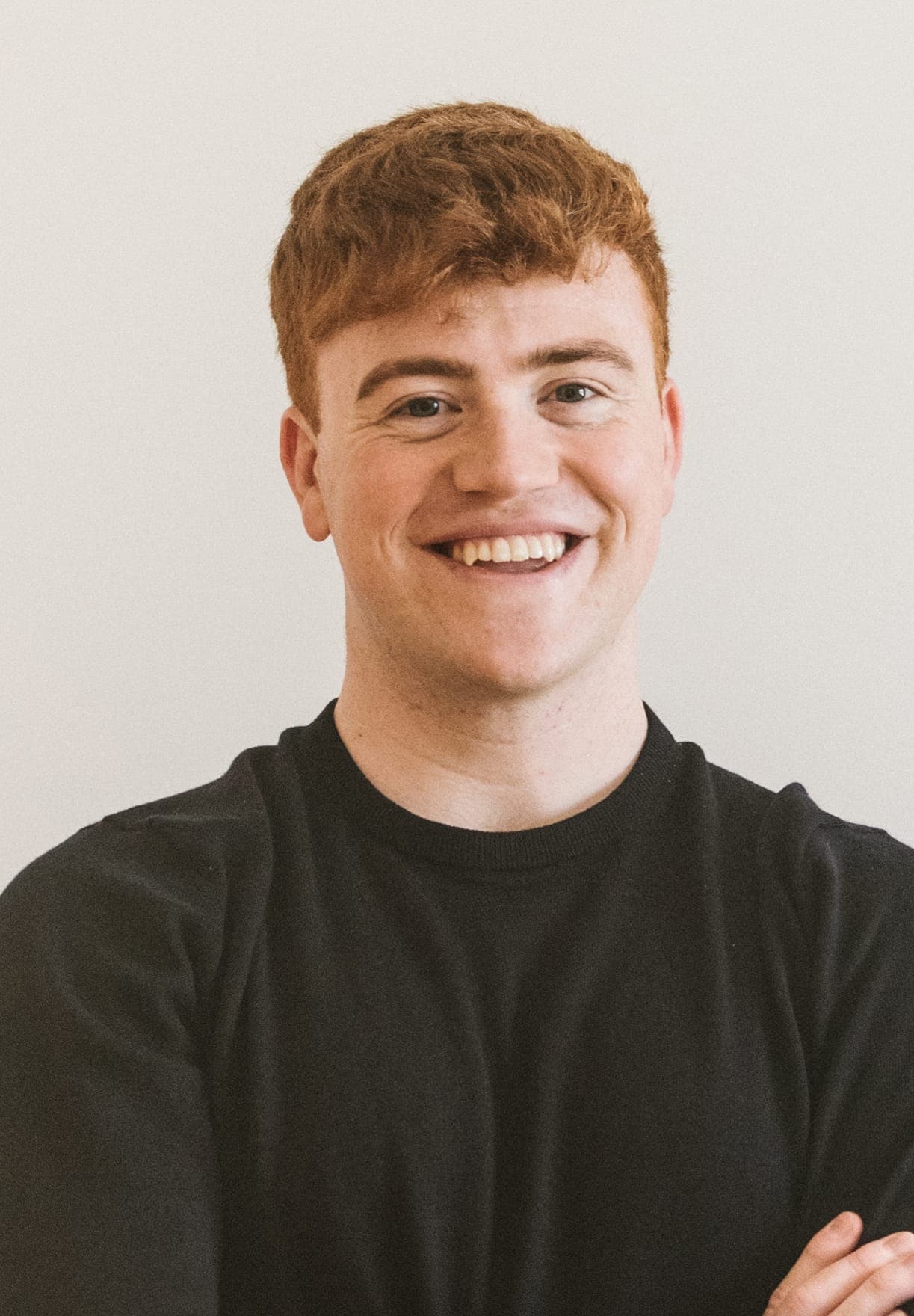