Agents
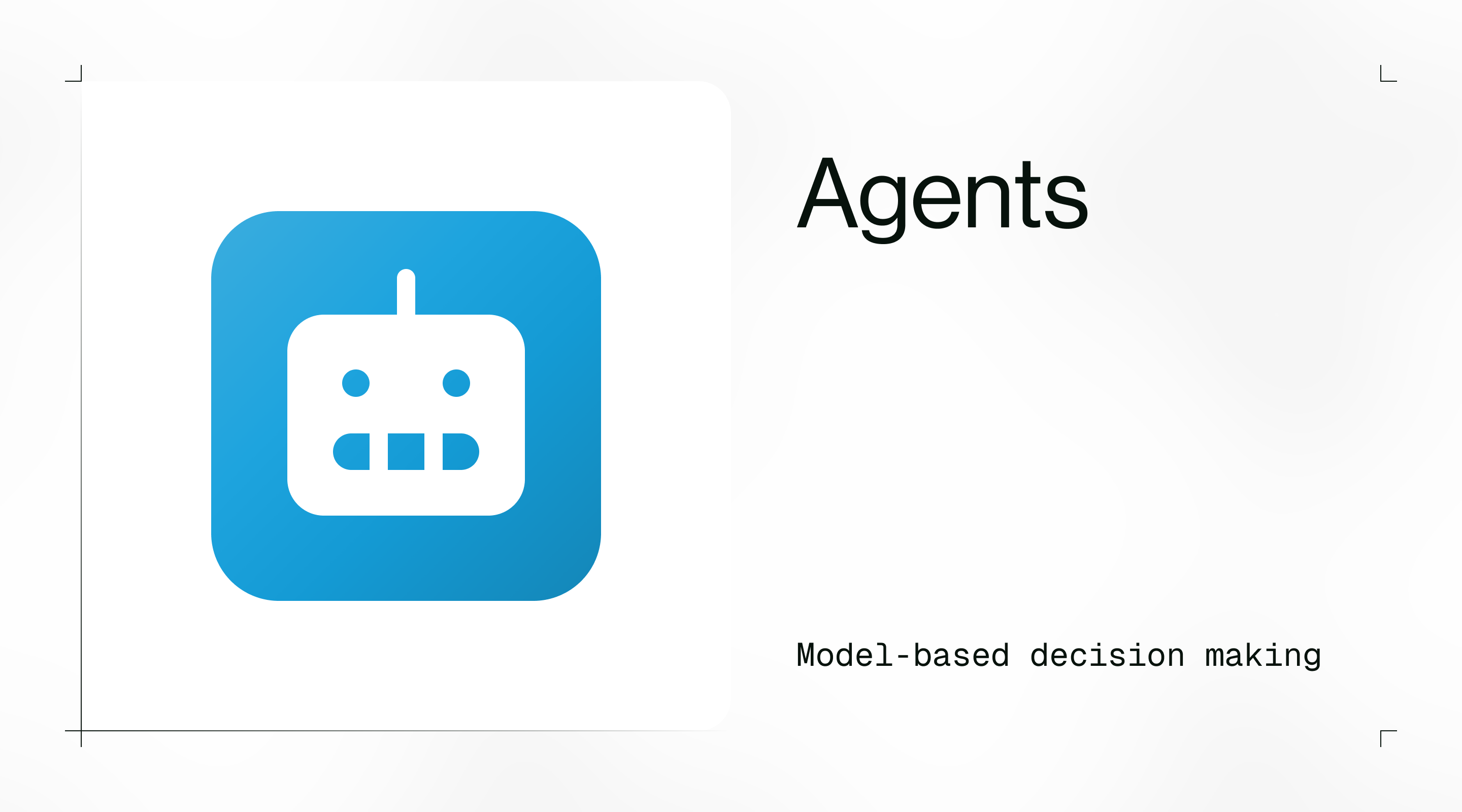
Agents are currently in beta and subject to change.
Overview
A Humanloop Agent is a multi-step AI system that leverages an LLM, external information sources and tool calling to accomplish complex tasks automatically. It comprises a main orchestrator LLM that utilizes tools to accomplish its task.
An Agent is composed primarily of:
- A template that instructs the Agent model how to process tasks
- Model parameters such as
temperature
,max_tokens
, andreasoning_effort
- The workflow configuration that defines how the Agent processes tasks, such as
max_iterations
andstopping_tools
- The available tools the Agent can use, including Tools and Prompts
An Agent executes on the Humanloop platform: for a given Agent version, if you supply the necessary inputs, it will execute until it reaches a stopping condition.
Humanloop will return a Log with an output
or output_message
, along with the full trace of the Agent’s execution.
Inputs are defined through variables in the Agent template. The values of these variables will need to be supplied when you call the Agent to create a generation. The Agent configuration and runtime data will be stored in Logs, which can then be used to create Datasets for evaluation purposes.
Basics
You can create and configure Agents through the Humanloop UI or programmatically via our SDK.
Agents in the UI
To create an Agent, create a new file and select the Agent option.
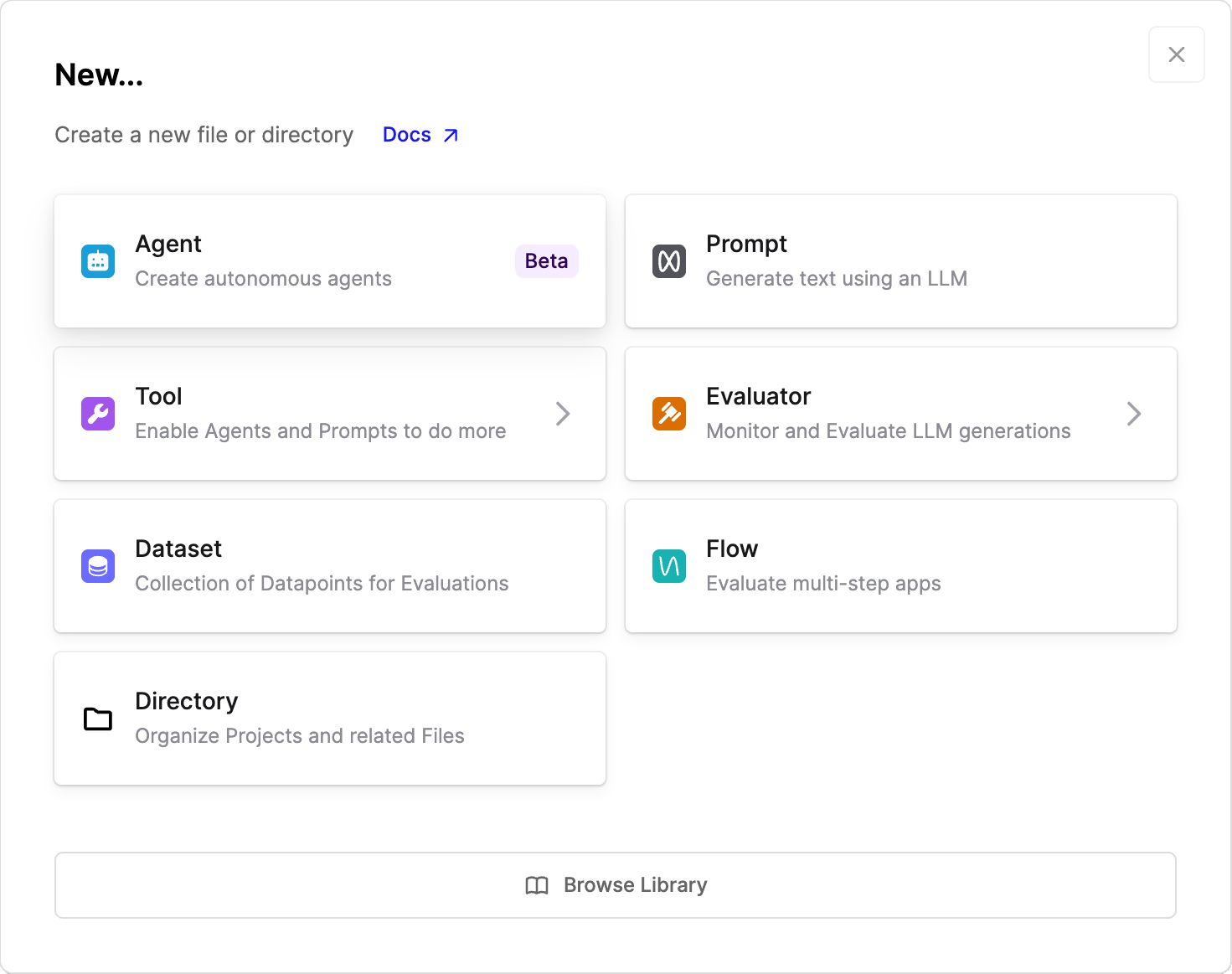
Create a new Agent file.
Then, use the Agent Editor to configure and test the Agent. You can link existing Tools and Prompts from your workspace for the Agent to use. You can also define a tool inline by providing its JSON schema. You can also configure the max_iterations
and stopping_tools
for the Agent in the “Parameters” section.
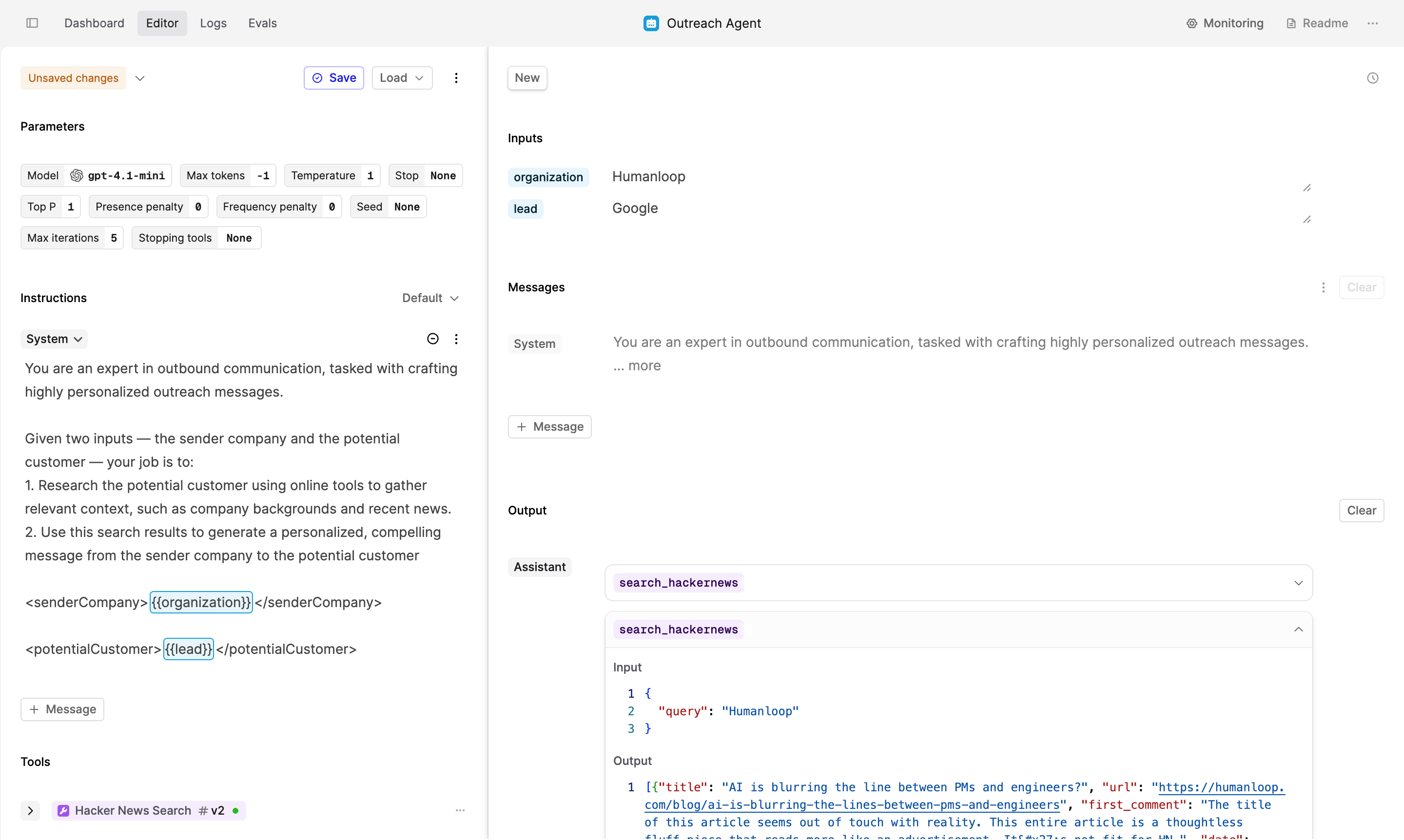
Agents via the SDK
To create an Agent programmatically, use our SDK.
Agents can be called through our UI for testing and development, or via API for production deployments.
If your Agent calls a tool that Humanloop cannot run, the system will pause and wait until you provide the response required by the tool. You can then continue the Agent’s execution using the /continue
endpoint.
You can also log Agent executions explicitly when running the Agent logic yourself:
Versioning
Versioning your Agents enables you to track how adjustments to template, tools, or model parameters influence the Agent’s behavior. This is crucial for iterative development, as you can pinpoint which configuration produces the most effective results for your use cases. You can use the Editor or SDK to create and update Agent Versions and iterate on improving its performance.
The following components of an Agent contribute to its version:
- The Agent’s template — the system prompt and any other instructions
- The model call parameters — such as
temperature
,max_tokens
, andreasoning_effort
- The Agent’s workflow configuration — such as
max_iterations
andstopping_tools
- The Agent’s tools — including both inline JSON Schema tools and linked Tool or Prompt Versions
Stopping Conditions
An Agent will stop executing in three circumstances:
- The maximum number of iterations is reached
- The Agent executes a tool that is configured to stop the Agent
- The Agent has generated a response with no further tool calls
Max Iterations
You can cap the maximum number of iterations an Agent can execute—the Agent will generate up to max_iterations
times, and then stop.
This results in an Agent Log with a finish_reason
of "max_iterations_reached"
.
Stopping Tools
You can designate that a tool should stop the Agent after it is called. If it can be run on Humanloop, it will be run and the result logged before the Agent stops.
This results in an Agent Log with a finish_reason
of "stopping_tool_called"
and stopping_tool_names
populated.
Agent Finished
Lastly, the Agent will stop if it has generated a response with no further tool calls. This represents a natural end to the Agent’s execution, and results in an Agent Log with a finish_reason
of "agent_generation_finished"
.
Evaluation
Agents can be evaluated both through the Humanloop UI and programmatically. After each Agent execution, configured evaluators will run automatically on Humanloop.
Get started with evaluating your Agents in the UI or programmatically.
Tracing
A trace is the collection of Logs associated with an Agent execution, showing the complete path from input to output, including all intermediate steps.
Every Agent trace includes:
- The initial input and messages, and final output
- All intermediate LLM calls and their responses as nested Logs
- Tools called by the Agent and their inputs and outputs as nested Logs
- Any errors or unexpected behaviors
- Timing and performance metrics
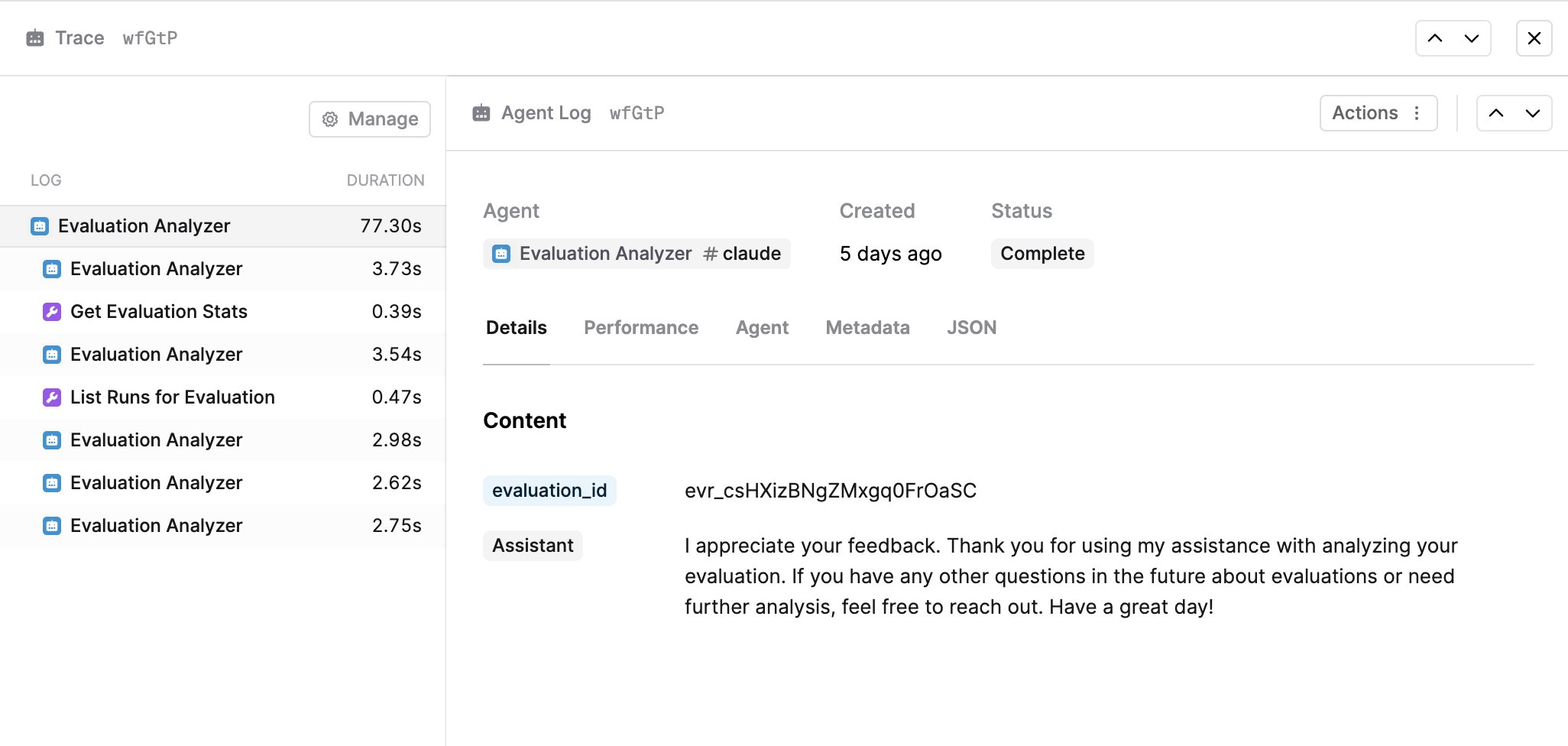
Metrics and Timing
Cost, latency, execution time, and token usage metrics are captured for each trace. They span from the earliest interaction to the final response.
Serialization
The .agent file format is a serialized representation of an Agent Version, designed to be human-readable and suitable for integration into version control systems alongside code.
The format is heavily inspired by MDX, with model and parameters specified in a YAML header alongside JSX-style syntax for chat templates.
Next steps
You now understand the role of Agents in the Humanloop ecosystem. Explore the following resources to apply Agents to your AI project:
- See our tutorials to learn how to evaluate your Agent via UI or via code.
- Explore our Tools documentation to understand what capabilities you can give your Agents.